Chapter 50
Animating Scroll View Using SwiftUI
In the earlier chapter, we introduced a new feature of ScrollView
in iOS 17, which enables developers to easily detect the scroll position and implement the scroll-up (or scroll-down) feature. In addition to this functionality, the latest version of SwiftUI also introduces a new modifier called scrollTransition
that allows us to observe the transition of views and apply various animated effects.
Previously, we built a basic scroll view. Let's continue using it as an example. For reference, here is the code for creating a scroll view:
ScrollView {
LazyVStack(spacing: 10) {
ForEach(0...50, id: \.self) { index in
bgColors[index % 5]
.frame(height: 100)
.overlay {
Text("\(index)")
.foregroundStyle(.white)
.font(.system(.title, weight: .bold))
}
.onTapGesture {
withAnimation {
scrollID = 0
}
}
}
}
.scrollTargetLayout()
}
.contentMargins(10.0, for: .scrollContent)
.scrollPosition(id: $scrollID)
Using ScrollTransition Modifier
A transition in scroll views describes the changes a child view should undergo when its appearing or disappearing. The new scrollTransition
modifier enables us to monitor these transitions and apply different visual and animated effects accordingly.
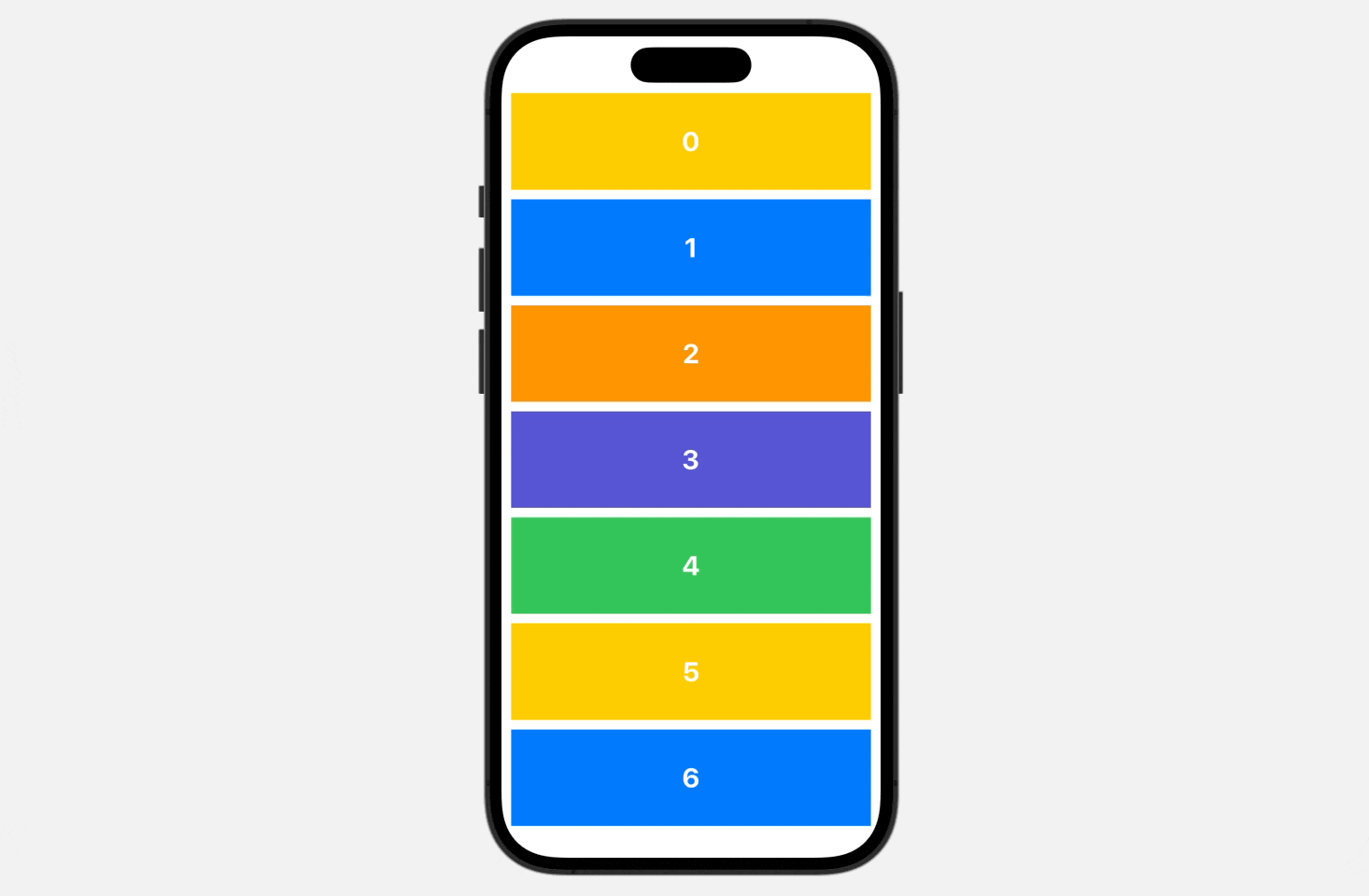
To demonstrate how it works, let's modify the code from the previous section by adding the scrollTransition
modifier to the color view. Here is the updated code:
To access the full content and the complete source code, please get your copy at https://www.appcoda.com/swiftui.