iOS Programming Basic: How Does the Hello World App Work?
I hope you enjoy the first iOS programming tutorial and already created your first app. Before we move onto the next tutorial and build a more complex app, let’s step back and have a closer look at the Hello World app. It’ll be good for you to understand some of the Objective-C syntax and the inner workings of the app.
So far you follow the step-by-step guide to build the Hello World app. But as you go through the tutorial, you may come across these questions:
- What are those .xib, .h and .m file?
- What are those “ugly” code inside “showMessage”? What do they mean?!
- What actually happens after you taps the “Hello World” button? How does the button trigger the “showMessage” action?
- How does the “Run” button in Xcode work?
I want you to focus on exploring the Xcode environment so I didn’t explain any of the above in the previous post. Yet it’s essential for every developer to understand the inner details behind the code and grasp the basic concept of iOS programming. For some technical concepts, they may be a bit hard to understand particularly you have no programming background. Don’t worry, however. This is just a start. As you move on and write more code in later tutorials, you’ll get better understanding about iOS programming. Just try your best to learn as much as possible.
Interface Builder, Header and Implementation Files
First, what are those .xib, .h and .m files? This is a very good question raised by one of the readers. Under the Project Navigator, you should find three main types of files – .xib, .h and .m. (If you expand the “Supporting Files” folder, you’ll find other file types such as plist and framework. But for now, let’s forget about them first. We’ll talk about them later.)
.xib – For files with .xib extension, they’re Interface Builder files that store the application’s user interface (UI). As you click on the .xib file, Xcode automatically switches to the Interface Builder for you to edit the UI of the app via drag-and-drop.

Interface Builder in Xcode
.h and .m – Files with .h extension refers to the header files while those with .m extension are the implementation files. Like most of the programming languages, the source code of Objective-C is divided into two parts: interface and implementation.
Well, to put in analogy that you can better understand both terms, let’s consider a TV remote. It’s convenient to control the volume of a TV set wirelessly with a remote. To increase the speaker volume, you press the “Volume +” button. To switch channel, you simply key in the channel number. Let me ask you. Do you know what happens behind the scene when pressing the “Volume” button? Probably not. I believe most of us don’t know how the remote communicates with the TV set and controls the speaker volume. What we just know is, that button is used for changing the volume. In this example, the button that interacts with you is the “interface” and the inner detail which is hidden behind the button is referred as the “implementation”.
Now you should have a better idea about interface and implementation. Let’s go back to the code. In Objective-C, interfaces of a class are organized in “.h” file. We use the syntax “@interface” to declare the interface of a class. Take a look at the HelloWorldViewController.h, which is the header file:
1 2 3 4 5 |
@interface HelloWorldViewController : UIViewController -(IBAction)showMessage; @end |
It starts with “@interface” followed by HelloWorldViewController, which is the class name. Inside, it declares a “showMessage” action, which is known as a method call.
Like the “Volume” button, apparently we do not know how the “showMessage” action works. You just know it’s used to display a message on screen. The actual implementation is put in the HelloWorldViewController.m, the implementation file:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
@implementation HelloWorldViewController // I've removed other methods for better reading. Focus on the showMessage method first. - (IBAction)showMessage { UIAlertView *helloWorldAlert = [[UIAlertView alloc] initWithTitle:@"My First App" message:@"Hello, World!" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil]; // Display the Hello World Message [helloWorldAlert show]; } @end |
As you can see from the above, you use the declaration “@implementation” to declare an implementation. Inside the “showMessage”, it’s the actual code defined to display the alert message on screen. You may not understand every single line of code inside the “showMessage” method. In brief, it creates an UIAlertView with “My First App” as the title and “Hello, World” as the message. It then calls up the “show” method and request iOS to display the pop-up message on screen.
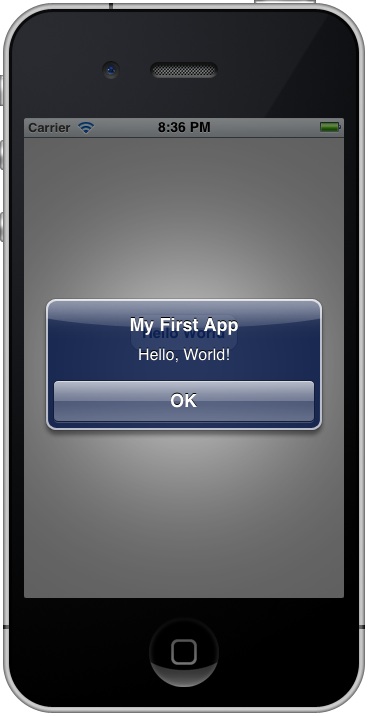
Hello World App
It’s vital for you to understand the concept of interface and implementation. If you have any question, feel free to raise your question at our forum.
Behind the Touch and Tap
What actually happened after tapping the “Hello World” button? How does the “Hello World” button invoke the “showMessage” method to display the “Hello World” message?
Recalled that you established a connection between the “Hello World” button and the “sendMessage” action in Interface Builder. Try opening up the “HelloWorldViewController.xib” again and select the “Hello World” button. Click the “Sent Events” button in the Utility area to open the Sent Events.
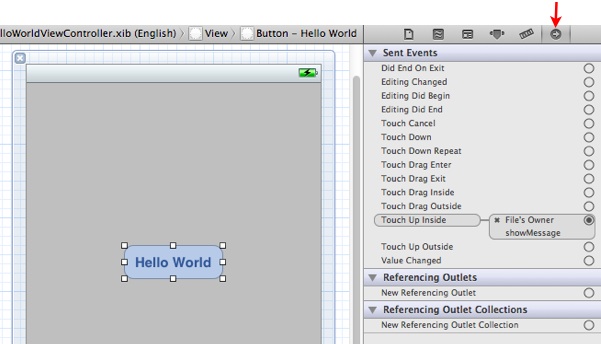
The Sent Events section shows you all connections between events and actions. As you can see in the above figure, the “Touch Up Inside” event is connected to the “showMessage” action. In iOS, apps are event-driven. The control/object (e.g. UIButton) listens for certain events (e.g. touches and taps). When the event is triggered, the object calls up the preset action that associates with the event.
In our Hello World app, when users lift up the finger inside the button, the “Touch Up Inside” event is triggered. Consequently, it calls up the “showMessage” action to display the “Hello World” message.
The below illustration sums up the event flow and what I have just described.

Event and Message Flow of Hello World App
Behind the Scene of the “Run” Button
When you click the “Run” button, Xcode automatically launches the Simulator and runs your app. But what happens behind the scene? As a programmer, you have to look into the entire process.

The entire process can be broken into three phases: compile, package and run.
Compile – You probably think iOS understands Objective-C code. In reality, iOS only reads machine code. The Objective-C code is only for you, the programmer to write and read. To make iOS understand the source code of the app, we have to go through a translation process to translate the Objective-C code into machine code. This process is referred as “compile”. Xcode already comes with a built-in compiler to compile the source code.
Package – Other than source code, an app usually contains resource files such as images, text files, xib files, etc. All these resources are packaged to make up the final app.
We used to refer these two processes as the “build” process.
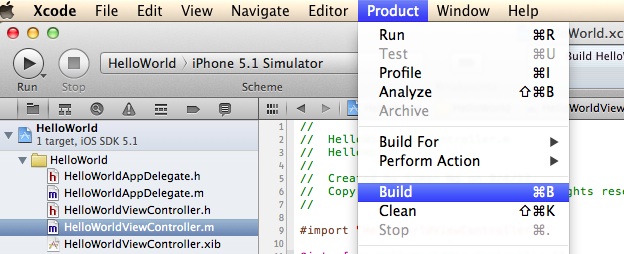
Run – This actually launches the Simulator and loads your app.
Got Questions?
I try my best to explain how Hello World app actually works. As a beginner without prior programming experience, it’s not easy to understand all the concepts we just discussed. So don’t hesitate to ask questions at our forum. I, as well as, other experienced members are eager to help.
As always, leave me comment to share your thought about the tutorial. Comments are always welcome. π
Comments
Alan Tong
AuthorGreat! I like the TV remote analogy.
Simon Ng
AuthorThanks, Alan.
Tom
AuthorI agree, great analogy!
Keep up the great work π
Simon Ng
AuthorThanks! Great to hear you also like the analogy.
William
AuthorNice analogy, really appreciate you breaking down the different parts of the project and the Xcode workspace Looking forward to the second tutorial!
Simon Ng
AuthorThanks! I’m now working on the next tutorial. It should be too far away.
SimonZA
AuthorNice follow-up to the first tutorial!
Quick question, we’ve spoken about the .xib, .m and .h files. The two other files that are produced called ‘…delegate.m’and ‘…delegate.h’, how important are those? Do we need to worry about them? And what point in app creation would we need to become familiar with those, if at all?
Thanks again! π Loving your work!
Simon Ng
AuthorDon’t worry about the AppDelegate class. But in brief, it’s the delegate of UIApplication. If you’ve looked into the class, you’ll find methods such as applicationDidEnterBackground, applicationWillTerminate. There is no implementation by default. Say, you’re developing a game, you’d like to save all the game data before your app is terminated. You may put the code inside the “applicationWillTerminate” method. iOS automatically invokes this method in when your app is about to terminate. For details about the AppDelegate, you can refer to Apple’s official API doc.
Tekle
AuthorHi Simon,
Thank you for the two great tutorials on iOS programming done up so far. I am looking forwards to the next one.
Like the others, I also liked the analogy about the TV remote. It helped clarify a lot. But, that also triggered another question in my mind. It is about creating the connection between the “Hello World” button and the “showMessage” action by dragging from the button to the file’s owner. What has happened after we did that? Obviously, some code must have been written some where.
In the second tutorial, you showed us the different “Sent Events” and the “Touch Up Inside” is shown linked to the “File’s owner showMessage” with the radio button of the “Touch Up Inside” selected, and that must have been the result of dragging the “Hello World” button to the “showMessage” action.
So, in terms of code, what was written and where? Or am I trying to put the cart before the horse? May be you will bring us to that in due course after we have covered more ground.
Thank you.
Tekle
pdwalker
AuthorTekle,
To your first question, open up the xib file in a text editor. Not only does it define the user interface, it also contains a description of what methods and what view controller classes are driven by what elements.
The interface builder takes care of all that crap for you so you don’t have to write any of that horrible xml. Still, it’s nice to be able to look into the xib files and understand a little of what is actually happening behind the scenes.
I believe your second question is also answered the same way as the first. The events are described in the xib file I believe. Change the event tied to the sendMessage method and then compare the xib file with the original. Do you see if there is a difference?
Skinnypinny
AuthorThanks so much for your tutorials! They’re really easy to understand and follow!
MJun
AuthorThank you so much, wished I found you earlier : )
Ana
Authorthank you thank you thank you so much for the tutorials! they are the best ones! π
ani4may
Authoryou ROCK !
Midhun Raj
Authorthanks alot…!! .i jus made my first i phone app!!!
Chaaruu
AuthorThis tutorial is really very good for beginner…Thank you very much for this tutorial.
scaryme
Authori felt its the best tutorial to start with….felt great making my first app…keep coming up with more of such tutorials..thank u so much
Simon Ng
AuthorGreat to hear that! You can always check out the free tutorials here: http://104.131.120.244/ios-programming-course/
Nidhi
AuthorJust loved the details you have given with the screenshots and I can very confidently say this is the best tutorial to start with.
Good work dude. Cheers!
R. George
AuthorThis tutorial is really helpful, just what I need to get into iOS programming. Thanks so much!
JW
AuthorWow! This is a great beginner tutorial. Simple and get to the point with screenshot. My I.Q. went up by a point! I am going to read the rest of the tutorials. Thank you for taking the time creating this web sites.
bob
AuthorVery helpful tutorial Thanks
Make more
Cool Tim
AuthorYes! Make more!
khanhtungna
AuthorVery helpful guide. Thank so much!
Ram
Authoramazing tutorial
Shailesh
AuthorSuperb tutorial π You have explained very nice way. Thank you very much π
phuctrantuan
Authorit’s very easy to understand. Thank you so much.
Nabeel
AuthorYou have given the best details……..thanks
Aakash
AuthorIt’s make easy to understand about the flow. Really good tutorial.Thanks
Jacob
AuthorHow kind you are!. Awesome!
Ben Taylor
AuthorThanks Simon, you explained the tutorial very well and it was easy to follow and execute.
Appreciate your effort!
Simon Ng
AuthorGreat to hear that!
Prasanna
AuthorAll your tutorials are awesome. As as beginner, I could easily understand and learn iOS App. Thanks.
Nethra
AuthorThanks a lot.. .!!!! 2 days before i’ve got appointed as iphone developer in a company, which doesn’t provide any training but gives time to learn our self. your tutorial helping me alot to start my journey as a hardcore iphone developer…:-)
Untitled Entitled
AuthorLoving your tutorials.
kostino
Authorvery helpful tutorial thanks to the guy who made it ..just one question… i know C++ really well is objective c as difficult to learn or it is more difficult?
Simon Ng
AuthorIf you already have C++ background, it shouldn’t be difficult for you.
Sandip Pund
AuthorAmazing Tutorial for Beginners…..Good Work…!
Carlos
AuthorI would love to see this on XCode 4.6
Yogesh
AuthorNow after referring to dozens of online tutorials I can say, I found what I was looking for π Thanks, for the awesome explanation and sharing knowledge.
sonnt
AuthorThis tutorial is so helpful!
In x-code 4.5, did .xib file was replaced by .storyboard file?
Simon Ng
AuthorIt doesn’t replace .xib. You are free to choose from Interface Builder or Storyboard. If you want to learn more about Storyboard, you can check out this tutorial:
http://104.131.120.244/use-storyboards-to-build-navigation-controller-and-table-view/
Hanne
AuthorThank you, this is very helpful π
obloodyhell
AuthorOK, anyone want to tell me how to END the app? I mean, it seems remarkably bad behavior to build this app that doesn’t just shut itself off when you are done with it — no, I don’t want to hit the XCode “stop” and chop it off at the legs — I want to tell the APP it is done INSIDE the app, and that it can close. :-S
No one seems to think this is something a beginner ought to be doing… lovely.
Chris
AuthorThis is not an oversight. It is also not something that ANY developer, beginner or otherwise, ought to be doing.
iOS apps, by design, don’t have an exit() or die() call that the developer explicitly makes.
iOS apps continue to run in the background until iOS determines that the OS is running low on resources. Then the app is ended by the OS. One can place some calls in the ApplicationWillTerminate (as of iOS 4; I assume it’s still called this) hook to take care of last-minute QUICK tasks (e.g. saving prefs/data to a .plist, etc.). If you take too long to complete these tasks, the app will be closed anyway.
If you want to close an app as a user, you can leave the app, double-home-button click, and then swipe up on the app (iOS 7+) or long-press and click the X that overlays the app (< iOS7).
As far as programmatically closing the app, this doesn't fall to the developer within the application lifecycle.
gyesy
AuthorThanks alot!!!
raj
Authoramazing , want some more examples ..
MTagb
AuthorI have been working with iOS dev for over a year, but loved this one.
Pat
AuthorFirst xcode tut i have been able to follow and get a working app out of it. so kudos!
helemi
Authorgreat job
Louie
AuthorGreat Interface and Implementation explanation. I like the level of detail you are teaching, not too much and not to few. A great kick starter.
Salophone
Authorawesome tutorial. Detail and clear explaination.. thanks.
CEMSOFT SOFTWARE
Authorthank you ; very useful tutorial for me.
Gabriel
AuthorVery good tutorial and explanation!!!! Thanks a lot!!!
Ste
AuthorThanks so much for this great tutorial. I’m a complete newbie to this and I’m really excited at how accessible iOS app development can be. It wouldn’t be without your help so I would like to thank you for putting your time and effort into helping people like me realise their dreams of writing their own apps. I don’t even care if my ideas are not original, I am just happy to have learnt these skills and to have apps on my phone which I can say I have coded (with help of course π ). Thanks
Kumar Subramani
AuthorThanks Simon..
ROONEY
AuthorThanks a lot, Simon. You make it so simple, and easy to learn.
Hoping to see more how-to articles in future.
Nick D
AuthorGreat tutorial, thanks a lot!
Arnab Bose
AuthorThanks for your tutorial.It’s very easy to understand. Thank you so much.
Maulik
Authorwhat is the extension of file/package it creates once the “build” is finished… i mean is it .exe file??
GrayChen
AuthorI like ur tutorial.
joseph
Authorsuper thanks
Mulie
AuthorThank you very much for your kind contributions and for doing your best in all lessons!
Saran
AuthorAs a beginner,this tutorial helped me a lot.Thank U
shilpa
Authoru are just awesome… thank u so much..:) :*
Vinicius de Paula
AuthorExcellent teaching, congratulations!!! π
Bailey
AuthorBest Tutorial I have found, and its free!
waye
AuthorI can not find any tutorials better than yours!
Nikola KneeJah Markovic
AuthorBest. Class. EVER!
maikytec
AuthorExcelent…
Vaishali Modi
AuthorNice tutorial for beginners. Thanks.. π
Bhavin Kansagara
AuthorBest Explained with the image of entire process happening behind the events…Really appreciated..Thanks
Tam
AuthorThe best tutorial Ive ever seen
smilealgernon
AuthorHello,i have a question.I find the xib is xml formatοΌI was wondering how does xcode deal with the xml file when compiled. sorry about my english…
Rubim Shrestha
Authorgreat tutorial…. (Y)
Mathew A
Authorgreat tutorial…but in what case do i have to use the .xib or storyboard ? i don’t understand the difference between them
fizza
AuthorJust awesome explanation!!!
Sanjeev Reddy
AuthorReally Awesome .Really from scratch.I enjoyed it.
Ashish
AuthorConcise and Clear. Great Work
gaganglobin76
AuthorVery good and clear explanation about building first app in iOS
Shasvat
AuthorAs a beginner, I think i can’t better tutorials like appcoda!!!
Shasvat
AuthorAs a beginner, I think i can’t get better tutorials than appcoda!!!
Prakash
AuthorI am very thankful to you. Thanks for your great work π
bmccarron
AuthorNicely done. I finally feel comfortable enough to begin. Thank you.
Oleg
AuthorThe best tutorial I found so far π
sunil
Author@interface helloworldviewcontroller : UIViewController
is it means that interface inheriting UIViewController….????
KanaanRaza
Authoryes, your custom ViewController “helloworldviewcontroller” class is being inherited from UIViewController.
sunil
Authorin .h file
#import
what it means?
in .m file
#import “some.h”
what it means??
sunil
Authorwhat is appdelegate..? and what is viewcontroller??
in HelloWorld Example
appdelegate .m file contains only @implementation ……… @end
where as
viewcontroller .m file contains @implementation ………. @end
and also this
@interface HelloWorldViewController ()
@end
what it means??
bremmi
Authorgood explanation..!
karthik
Authorthanks Dear sir
mpred
AuthorF****** amazing!!! It’s clear, simple, direct. Love it! Bring us some more pls!
Kyle
AuthorThank you so much this was an amazing tutorial!
tknvx
AuthorThanks!
Dhiraj
AuthorAwesome Explanation as i am looking for this. Thanks……….
Hamidreza GHaderi
Authortnx Simon
Khushboo
AuthorThnQ very much!!!!This is really helpful and u make objective c interesting!!:)thnq again
Nishant Malhotra
AuthorIts indeed a good startup tutorial, to begin with . Just a question how to deploy the hello world app to my iphone
princeashok
Authorreally helpful tutorial….
i need some more clarity from you..
1. what is IBAction?
2. You are creating instance of UIAlertview and I think initWithTitle, message, delegate, cancelButtonTitle and otherButtonTitles are all properties of UIAlertview right?
3. showMessage method has to return IBACtion right?if i am wrong please let me know..
thanq.. π
badtrack0
AuthorMany thanks. It’s great for me.
Chandan Singh
AuthorThank you so much…!! it was very informative and exciting the way you explained in tutorial. Actually I have good hand-on programming, So each and everything you explained was bit easy for to me catch up the concepts.
hari
Authorwhat happens when we click on build?
ravi
AuthorExcellent tutorial for beginners
Pratik Chawke
AuthorHi Simon,
Great Job !!! Best Tutorial i have found to learn ios. As a beginner this is the best way to get understand objective c. In latest Xcode .xib replaced with storyboard and found updated UIAlertController over UIAlertView. Thanks
Tilak
AuthorIt’s a very Nice walkthrough of basics. Would be interested in revisiting. π